If you are looking for information on how to get post by ID, all you need is to use the get_post() function. This article guides you through some specific cases on how to get post content by ID in WordPress and extended cases such as: get the title, get category name, get post date, get term by post id.
Table of Contents
- 1 5 examples of getting post by id
- 2 11 Ways to Get Post ID in WordPress
- 2.1 Post ID in WordPress Database
- 2.2 Add the Post ID column to the WordPress Posts Table
- 2.3 Get ID From the Global $post object
- 2.4 Using get_the_id() and the_id() functions
- 2.5 Get Post ID by Title, SLug
- 2.6 Get post ID from URL in WordPress
- 2.7 An easier way to get post ID in WP_Query loop
- 2.8 Get Post ID by meta key
- 2.9 Get Post ID by Term ID (Category ID, Tag ID)
- 2.10 In URL of the Post Without Custom Permalink Structure
- 2.11 In URL on the post edit page
- 2.12 Get Post ID of Static FrontPage
- 2.13 How to Get page id in WordPress?
- 2.14 How to get post ID by title in WordPress?
- 2.15 Get featured image post in WordPress by post id
- 2.16 How to get a post id from permalink?
5 examples of getting post by id
get post by ID in WordPress is a type of query to get information of one or more posts based on the post’s ID. Here are the 3 most common ways to get posts by ID in WP
Get post status by post id
There are many ways to get post status by post id, but the best way is to use the get_post_status function.
$current_post_status = get_post_status( 18 ); // 18 is post ID
Get post type by id
We can use the get_post_type function to Get post type by id:
$post_type = get_post_type( $id ) // $id is post ID if ( $post_type == 'post' ) { //if is true }
WordPress post content by ID
The first question is how can I get WordPress post content by post id? The way to do is quite simple is to use get_post() to get the data and then use apply_filters() to filter. Here is a sample example of getting post content by ID in WordPress
$my_post_id = 555;//Post ID $content_post = get_post($my_post_id); $content = $content_post->post_content; $content = apply_filters('the_content', $content); $content = str_replace(']]>', ']]>', $content); echo $content;
The same way to get specific page content. This is the proper way to get page content
<?php $id=47; $post = get_post($id); $content = apply_filters('the_content', $post->post_content); echo $content; ?>
The second way is to use WP_Query to display the content of a custom post type based on its ID.
In this example, I want to get the content from the post with ID 55.
There is a function in there to get the featured image URL.
The example below will display the title, featured image with size 1280×800, and the full content of the post.
<?php $my_id = 55; $args = array('p' => $my_id, 'post_type' => 'about'); $result = new WP_Query($args); while ( $result->have_posts() ) : $result->the_post(); ?> <?php global $post; ?> <?php the_title(); $src = wp_get_attachment_image_src( get_post_thumbnail_id($post->ID), array( 1280,800 ), false, '' ); ?> <div class="section" style="background: url(<?php echo $src[0]; ?>) no-repeat center center fixed; -webkit-background-size: cover; -moz-background-size: cover; -o-background-size: cover; background-size: cover; z-index:-1;"> <?php the_content () ?> <?php endwhile; ?>
Get post title and post content by ID
To get the title for a post with ID 100:
$post_query = get_post( 100 ); echo $post_query->post_title;
To get the post content for a post with ID 100:
$post_query = get_post( 100 ); echo $post_query->post_content;
The second way is to use the get_post_field() function to get the data
The syntax of this function:
get_post_field( $field, $post_id, $context );
So code, that will get the title by post-id 100 looks like this:
$post_title = get_post_field( 'post_title', 100 );
Because of that, you want to display the title, so you can ignore the last param
Similarly, you can get the post content from the post_content field
$title = get_post_field( 'post_content', 100 );
Here are the default post fields that you can get (case-sensitive):
ID post_author post_date post_date_gmt post_content post_title post_excerpt post_status comment_status ping_status post_password post_name to_ping pinged post_modified post_modified_gmt post_content_filtered post_parent guid menu_order post_type post_mime_type comment_count filter
The above methods can be applied to many different post_types such as post, page, or custom post type
Get posts by multiple post IDs?
You can use get_posts() as it takes the same arguments as WP_Query.
To pass it the IDs, we use ‘post__in’ => array(25,28,65,72,86) (remember to put it in the array).
Below is an example of getting content for posts with IDs of 25,28,65,72,86. Foreach loop is used here to run through all found values
$args = array( 'post__in' => array(25,28,65,72,86) ); $result_posts = get_posts( $args ); if( ! empty( $result_posts ) ){ $output = '<ul>'; foreach ( $result_posts as $p ){ $output .= '<li><a href="' . get_permalink( $p->ID ) . '">' . $p->post_title . '</a></li>'; } $output .= '</ul>'; } echo $output ?? '<strong>Sorry. No posts matching your criteria!</strong>';
The second way is to use WP_Query. Here is an example
<?php $args = array( 'post__in' => array(25,28,65,72,86), 'post_status' => 'publish', 'orderby’ => 'title', 'order’ => 'ASC', ); // The Query $the_query = new WP_Query( $args ); // The Loop if ( $the_query->have_posts() ) { echo '<ul>'; while ( $the_query->have_posts() ) { $the_query->the_post(); echo '<li>' . get_the_title() . '</li>'; } echo '</ul>'; } else { // no posts found } /* Restore original Post Data */ wp_reset_postdata(); ?>
The result will show out like this:
- Article Title 25
- Article Title 28
- Article Title 65
- Article Title 72
- Article Title 86
In short, there are 2 functions you can use to get post by ID, here are the 2 most basic examples to help you remember and practice
Method 1: use get_post() function, example:
$post = get_post( 55 ); // Get a post title by ID echo $post->post_title;
Method 2: Use WP_Query
Or you can get post by ID with WordPress WP_Query too:
$query = new WP_Query( array( 'p' => 55 ) ); $query->the_post(); // Get post type by ID echo $query->post->post_type; // Get post status by post ID echo $query->post->post_status; // Get post content by post ID echo $query->post->post_content;
11 Ways to Get Post ID in WordPress
Next, we continue to look in the opposite direction, how to get Post ID in WordPress?
There are many ways to do this, depending on the specific case. Below I introduce 11 methods to get post-id of a post or a page with specific situations
Post ID in WordPress Database
In WordPress’s database, there is a table named wp_posts (yours may be different because the prefix of each website is different). When you click on the wp_posts table to view the information, there is a column called the ID column. You can find the Post ID of any post/page there
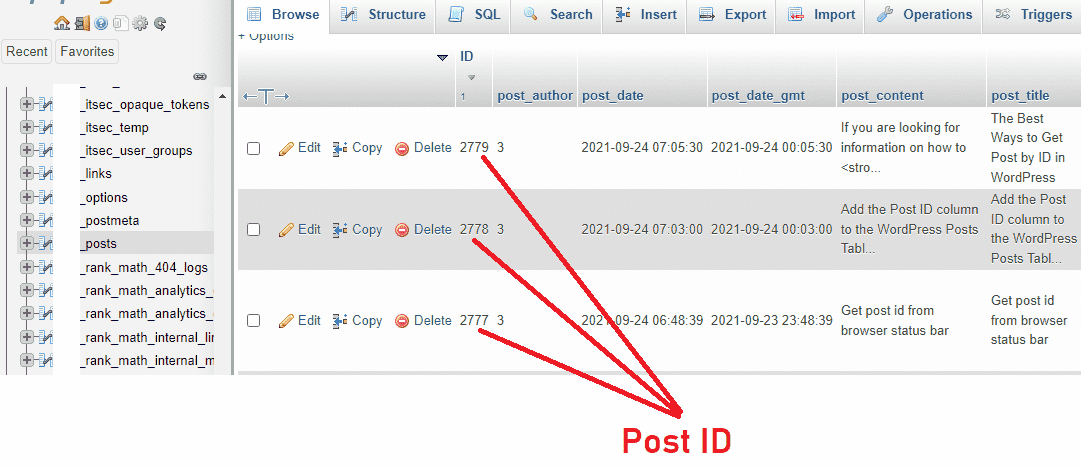
Add the Post ID column to the WordPress Posts Table
This second method is more professional when you add an ID column of the article to the page displaying the list of posts in the admin area. This way is quite professional and convenient, I also recommend using this way to display the ID of each post in the admin area.
You also don’t need to know the code, because I already wrote the code below, your task is just to copy and paste it into the last line of the functions.php file in your theme.
/** * Add an empty column to the table displaying the list of posts in the Wordpress Admin area * * @param array $columns Array of all the current columns IDs and titles */ function hhpostid_add_column( $columns ){ $columns['huyhoa_postid_column'] = 'Post ID'; return $columns; } add_filter('manage_posts_columns', 'hhpostid_add_column', 5); //add_filter('manage_pages_columns', 'hhpostid_add_column', 5); // If you also want to display in the Page area, you can remove the 2 // signs at the beginning of this line /** * Display the ID of each article corresponding to the title of the post * * @param string $column ID of the column * @param integer $id Post ID */ function push_column_content( $column, $id ){ if( $column === 'huyhoa_postid_column') echo $id; } add_action('manage_posts_custom_column', 'push_column_content', 5, 2); //add_action('manage_pages_custom_column', 'push_column_content', 5, 2); // If you also want to display in the Page area, you can remove the 2 // signs at the beginning of this line
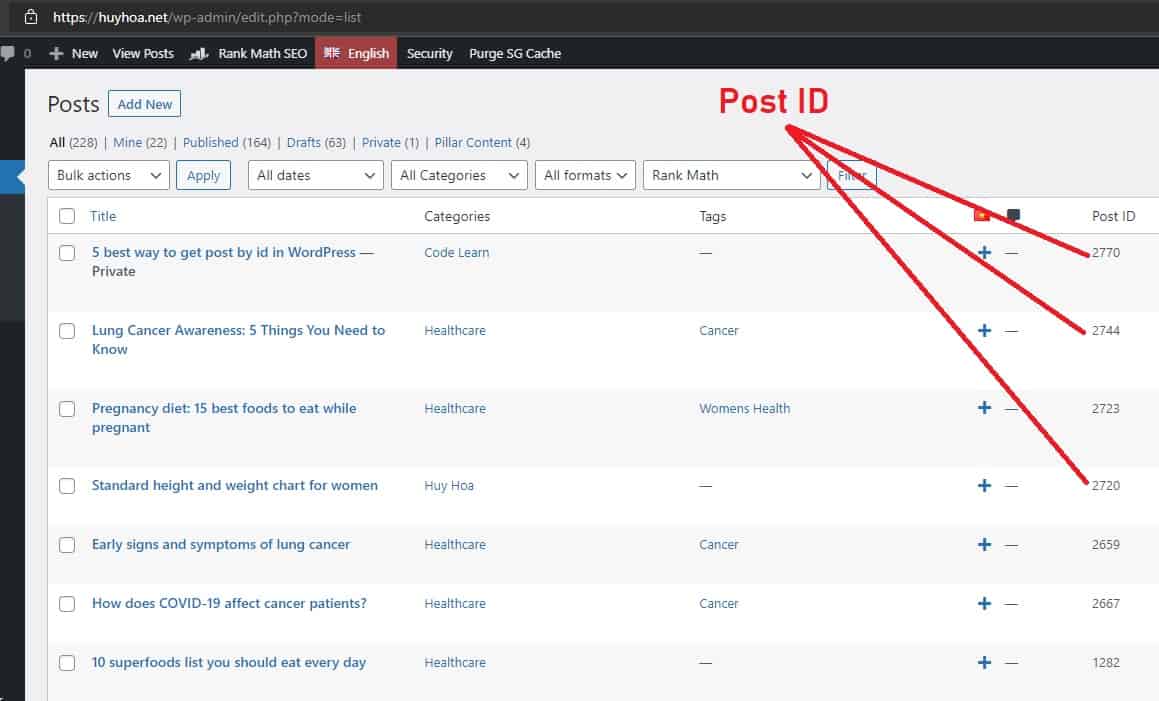
Get ID From the Global $post object
Note: The following examples all require some basic PHP knowledge. However, if you don’t know anything about the code, that’s okay, try and follow the detailed instructions below.
The $post global object contains all the data of the current post. The post ID is also present in $post, so getting the POST ID is pretty easy as we just need to output it:
global $post; echo $post->ID;
I use global $post so that if you use it in functions or plugins it can still display the POST ID
Using get_the_id() and the_id() functions
Both the get_the_id() and the_id() functions return the post’s ID. The difference here is that the get_the_id() function returns the numeric post’s ID and does not display it, while the_id() function will always display the post’s ID.
With the get_the_id() function, we have to echo the new ID to display:
echo get_the_id();
With the_id() function, we don’t need to echo because it’s already printed
the_id();
Get Post ID by Title, SLug
Get Post ID by Title
This is a built-in WordPress function and since version 3.0.0 it works not only for pages but also for any custom post type. You can use this function anywhere in your theme, plugins, or functions
$query_post = get_page_by_title( 'BEST WAY TO GET POST BY ID IN WORDPRESS', '', 'post' ); echo $query_post->ID;
Get Post ID by SLug
The function is similar to get_page_by_title(). You just need to replace the title with the URL slug. One note is that if your URL has both category or parent, you need to fill in either category or parent.
Eg:
$query_post = get_page_by_path('contact-us', '', 'post'); echo $query_post;
If the URL has a parent or a category, for example, /en/ao-dai/, you must fill in the URL completely.
$query_post = get_page_by_path('/en/ao-dai/', '', 'post'); echo $query_post;
Get post ID from URL in WordPress
To Get post ID from URL in WordPress, just use the code below. Replace the example URL below (https://huyhoa.net/wp-query/) with the URL you want to find the post’s ID
$post_id = url_to_postid( 'https://huyhoa.net/wp-query/' );
An easier way to get post ID in WP_Query loop
If you are inside the loop of WP_Query or any other loop, you can easily get the post ID by following way:
$query_post = new WP_Query( 'posts_per_page=5' ); while( $query_post->have_posts() ) : $query_post->the_post(); echo $query_post->post->ID; // print post ID endwhile;
Get Post ID by meta key
Actually, there are two ways to Get Post ID by meta key – that is using the WP_Query query or MySQL query. The below function I show you will return the post ID or an array of post IDs that match the given meta key.
function get_post_id_by_meta_key( $meta_key ) { global $wpdb; $ids = $wpdb->get_col( $wpdb->prepare( "SELECT post_id FROM $wpdb->postmeta WHERE meta_key = %s", $meta_key ) ); if( count( $ids ) > 1 ) { return $ids; // return array } else { return $ids[0]; // return int } }
Get Post ID both by meta key and value
It is similar to the previous function, but here you can also specify a meta value.
Just like Get Post ID both by meta key, the function below will get the id of the post that satisfies BOTH conditions is meta key and meta value
function get_post_id_by_metakey_and_value( $meta_key, $meta_value ) { global $wpdb; $ids = $wpdb->get_col( $wpdb->prepare( "SELECT post_id FROM $wpdb->postmeta WHERE meta_key = %s AND meta_value = %s", $meta_key, $meta_value ) ); if( count( $ids ) > 1 ) { return $ids; // return an array of Posts IDs } else { return $ids[0]; // return int of a Post ID } }
Get Post ID by Term ID (Category ID, Tag ID)
Here is an example function that gets Get Post ID by Term ID. The term here can be category id or tag id
function huyhoa_post_id_by_term_id( $term_id ) { global $wpdb; $ids = $wpdb->get_col( $wpdb->prepare( "SELECT object_id FROM $wpdb->term_relationships WHERE term_taxonomy_id = %d", $term_id ) ); if( count( $ids ) > 1 ) { return $ids; // return an array of Posts IDs } else { return $ids[0]; // return int of a Post ID } }
Usually, this function will return a multivalued array since the same term_id can have multiple articles attached to it. For example, with the same category id as 1, there can be hundreds of thousands of articles with the same topic. The same goes for tags.
In URL of the Post Without Custom Permalink Structure
To use this, you have to temporarily toggle your permalink settings in Settings > Permalink set to Plain. Now the p parameter in the URL is the post’s ID.
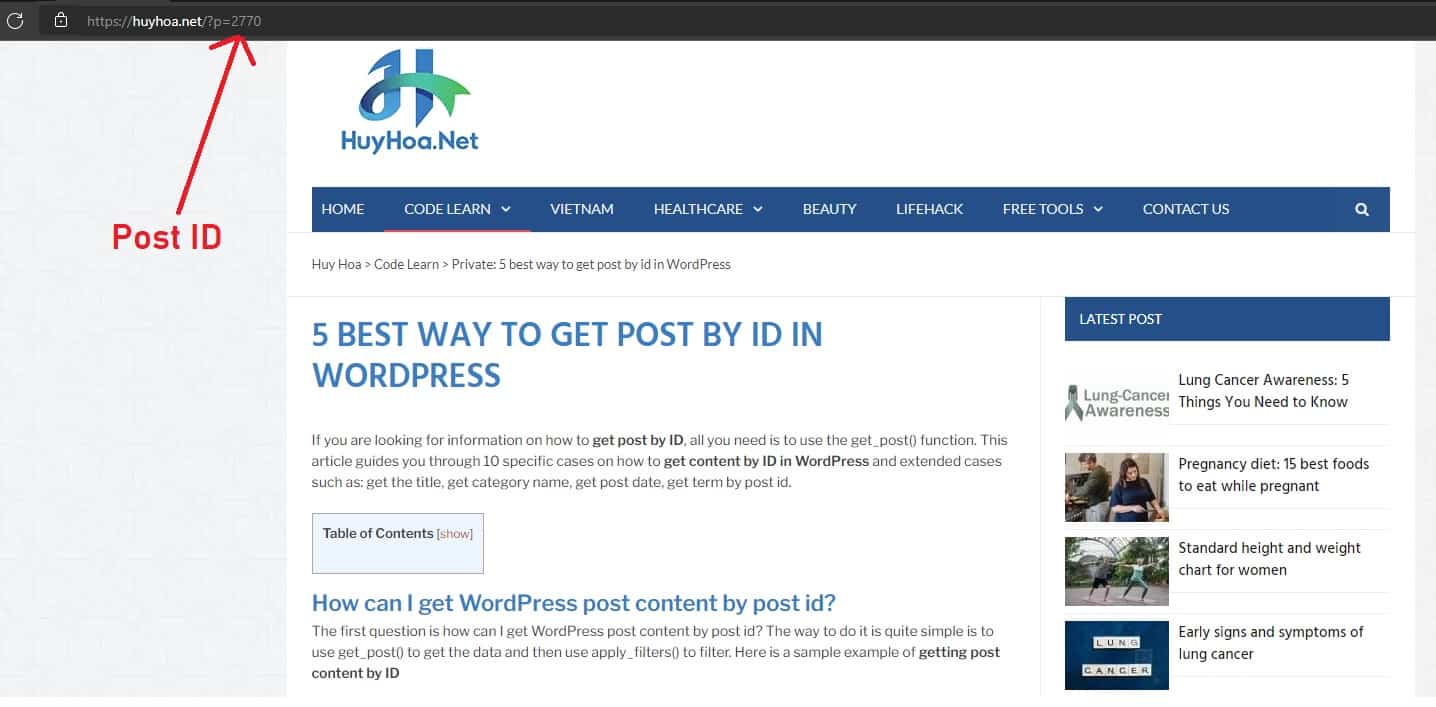
In URL on the post edit page
If you have access to post-editing, You can find the ID of the post in the URL when editing it.
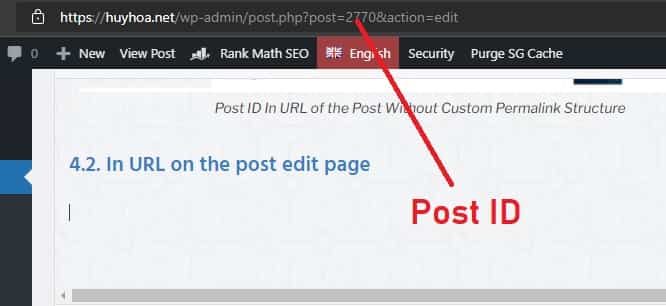
You don’t even need to open the post edit page. Just move your mouse over the «Edit» link or over the post title in the admin area and then look at the browser’s status bar (bottom left part of the screen) you will see the edit URL. edit including the post’s ID
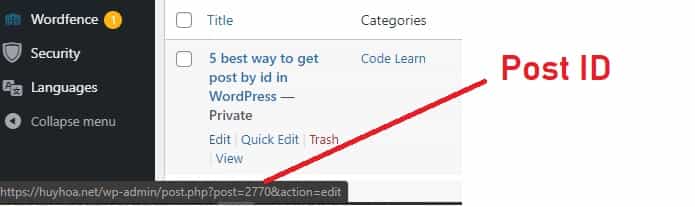
Get Post ID of Static FrontPage
A Static FrontPage is a specific Page used as the Home Page of the site.
If you want to get the ID of that static front page, you can use the following snippets to get the ID:
$frontpage_id = get_option( 'page_on_front' );
or get the ID of Posts page:
$blogpage_id = get_option( 'page_for_posts' );
Above are 10 ways to get posts by ID in WordPress. At the end of the article, I will show you some ways to get article information based on post id such as: get the post edit time, post time, get term by post id, get current URL.
How to Get page id in WordPress?
If in the page want to get the ID, then we can use the get_the_id() function:
$page_id = get_the_id();
Or we can use get_page_by_path() function as below:
$query_post = get_page_by_path('parent-page/sub-page', '', 'page'); $page_id = $query_post->ID;
We can find the $post array of a Page with the title of “World Peace Now”:
$query_post = get_page_by_title('World Peace Now', OBJECT, 'page'); $page_id = $query_post->ID;
How to get post ID by title in WordPress?
You can use the get_page_by_title() function to get the post ID based on the title
Examples are as follows:
$query = get_page_by_title('How to get the post ID by Title in Wordpress', '', 'post'); echo $query->ID; // Title is "How to get the post ID by Title in Wordpress"
Get featured image post in WordPress by post id
Here is the way to get a featured image of a post in WordPress by post id
<?php if (has_post_thumbnail( $post->ID ) ): ?> <?php $image = wp_get_attachment_image_src( get_post_thumbnail_id( $post->ID ), 'single-post-thumbnail' ); ?> <div id="featured-bg" style="background-image: url('<?php echo $image[0]; ?>')">
If you want to show it as an image instead of a background, then you can use this code:
<img src="<?php echo $image[0]; ?>" alt="<?php the_title(); ?>">
How to get a post id from permalink?
TO get the post-id from a permalink, you can use this function:
$post_ID = url_to_postid( 'https://huyhoa.net/en/get-post-by-id-wordpress/' ); echo 'Post ID = '.$post_ID;