You can completely customize the look or function of your sales page by editing the Woocommerce plugin directly, but writing custom code is always better. In this guide, I list the most commonly used ready-to-use custom WooCommerce functions that you can use to greatly increase the functionality of your online store to make your website stand out and bring give you a competitive advantage compared to other websites in the same industry and with the same function.
WooCommerce is an open-source plugin on WordPress that supports the design of e-commerce websites or sales websites. Its task is to turn your website into a fast and efficient e-commerce site.
Currently, about 13 million people are using WooCommerce for their website and about 37% of online shops operate on this plugin. So what makes WooCommerce so popular?
Here are some of the main reasons.
- WooCommerce is a free plugin.
- The installation and use method are relatively simple and easy. You don’t have to be a professional technician to do it.
- Provides a diverse library of WordPress templates, making the process of building an online shop homepage quick with just a few steps.
- Regularly updated with new features and supported by WordPress developers themselves.
- High security, helping to protect data, safe transaction information, prevent any illegal intrusion of third parties.
- WooCommerce allows users to configure with a very large number of built-in features or extensions.
- You can customize your shop, product, and cart pages easily through WooCommerce functions
To use these functions, you should create a child theme. After you have successfully set up your child theme, paste any of the code below into your child theme’s functions.php
file. You can place these code snippets at the bottom of the functions.php
file, just before the ?>
tag. If you don’t see the ?>
tag then just put it at the last line of your functions.php
Table of Contents
- 1 Where is the functions.php file in Woocommerce?
- 2 Renaming WooCommerce Product Tabs
- 3 Removing Tabs
- 4 Re-ordering Tabs
- 5 Add a custom tab
- 6 Move WooCommerce product additional information to Sidebar
- 7 Replace WooCommerce variable products price range with the min price
- 8 Replace the Variable Price range with the chosen variation price in WooCommerce 4+
- 9 Add Prefix / Suffix to WooCommerce Product Prices
- 10 Hide Prices on the Shop & Category Pages
- 11 Remove the “Default Sorting” Dropdown
- 12 Remove or Rename SALE! Badge
- 13 Replace “Choose an option” Text with Custom Text
- 14 Change “Total” text on WooCommerce Order confirmation template
- 15 Hide the “Add to Cart” button in WooCommerce
- 16 Adding Contact Form 7 to WooCommerce Product
- 17 Contact Form 7 + WooCommerce – Save form data in orders
- 18 Add Custom Product Fields in WooCommerce
- 19 Change Woocommerce “Thank you” message
- 20 Remove billing Address at WooCommerce Checkout Page
- 21 Remove Shipping address at WooCommerce Checkout Page
- 22 Reorder Checkout Fields in WooCommerce
- 23 Add Attributes to Short Description in WooCommerce
- 24 Hide SKUs on WooCommerce Product Page
- 25 Disable/hide Woocommerce single product page
- 26 Disable/hide Woocommerce category page
- 27 Get custom product attributes in Woocommerce
- 28 Display Contact Button if Woocommerce Product Price is zero
- 29 Add custom fields to WooCommerce registration form
- 30 WooCommerce show prices after login
Where is the functions.php file in Woocommerce?
Woocommerce does not have a functions.php file, it is a WordPress plugin. There may be some Woocommerce themes that may have a functions.php file but it’s not mandatory and when you insert the code into the functions.php file in the Woocommerce theme it won’t run either.
So, when I say “add this code to the functions.php file”, you must understand that is the active theme’s functions.php file. This functions.php file is usually located at wp-content/themes/your activated theme/functions.php
Here are the most commonly used code snippets that will help you customize Woocommerce functions and forms. These code snippets will help you to remove the default WooCommerce functionality or add new functionality to your WordPress Shopping page.
Renaming WooCommerce Product Tabs
If you want to change the title of the product tabs on the single product page, you can use the code below. The code below changes all 3 default tabs of Woocommerce from Description to Product Information, Additional Information to Extra Information, and Reviews to Product Reviews. If you don’t want to rename any tab, just remove that line in the code.
add_filter( 'WooCommerce_product_tabs', 'huyhoa_rename_tab', 98); function huyhoa_rename_tab($tabs) { $tabs['description']['title'] = 'Product Information'; $tabs['reviews']['title'] = 'Product Reviews'; $tabs['additional_information']['title'] = 'Extra Information'; return $tabs; }
Removing Tabs
Use the code below to remove any tab you want. The example below removes all 3 tabs on the single product page.
Note that when you want to keep a tab, delete the corresponding line to keep that tab.
add_filter( 'woocommerce_product_tabs', 'huyhoa_remove_product_tabs', 98 ); function huyhoa_remove_product_tabs( $tabs ) { unset( $tabs['description'] ); // Remove the description tab unset( $tabs['reviews'] ); // Remove the reviews tab unset( $tabs['additional_information'] ); // Remove the additional information tab return $tabs; }
Re-ordering Tabs
Use the following code to change the tab order. By default of WooCommerce, the order of tabs is Description, Reviews, Additional Information, respectively. The example below will move the Reviews tab first, then the Additional Information tab, and finally the Description tab.
/* * Reorder product data tabs */ add_filter( 'woocommerce_product_tabs', 'huyhoa_reorder_tabs', 98 ); function huyhoa_reorder_tabs( $tabs ) { $tabs['reviews']['priority'] = 5; // Reviews first $tabs['additional_information']['priority'] = 10; // Additional information second $tabs['description']['priority'] = 15; // Description third return $tabs; }
Add a custom tab
Use the following code to add a custom global product tab. This snippet will insert an extra tab on all products. This means that all products have an extra tab like that and the content is the same for all products.
/** * Add a custom product data tab */ add_filter( 'woocommerce_product_tabs', 'woo_new_product_tab' ); function woo_new_product_tab( $tabs ) { // Adds the new tab $tabs['test_tab'] = array( 'title' => __( 'New Product Tab', 'woocommerce' ), 'priority' => 50, // Show on last, you can set it to the first by change 50 to 5 'callback' => 'woo_new_product_tab_content' ); return $tabs; } function woo_new_product_tab_content() { // The new tab content echo '<h2>This is new tab</h2>'; echo '<p>Here\'s your new product tab. This tab will show on all product and this content is same for all product.</p>'; }
In case you want product A to display different content, product B to display different content, you can use the code below. In the example below, I take product A with id 11, product B has id 12. You can apply the same to other products or get by category.
/** * Add a custom product data tab */ add_filter( 'woocommerce_product_tabs', 'woo_new_product_tab' ); function woo_new_product_tab( $tabs ) { // Adds the new tab $tabs['test_tab'] = array( 'title' => __( 'New Product Tab', 'woocommerce' ), 'priority' => 50, // Show on last, you can set it to the first by change 50 to 5 'callback' => 'woo_new_product_tab_content' ); return $tabs; } function woo_new_product_tab_content() { // The new tab content global $product; if ($product->get_id() === 11) { echo '<h2>This is new tab for Product A with id = 11</h2>'; echo '<p>Here\'s your new product tab for Product A with id = 11. This tab will show on all product and this content is same for all product.</p>'; } elseif ($product->get_id() === 12) { echo '<h2>This is new tab for Product B with id = 12</h2>'; echo '<p>Here\'s your new product tab for Product B with id = 12. This tab will show on all product and this content is same for all product.</p>'; } else { echo '<h2>This is new tab for all other products</h2>'; echo '<p>Here\'s your new product tab for all other products. This tab will show on all product and this content is same for all product.</p>'; } }
The WooCommerce product additional information tab is where the Product attributes are displayed.
Actually, there is no DIRECT way to move the additional information tab to the sidebar, but we can do it INDIRECTLY by removing the additional information tab on a single product display page, then creating a shortcode to display information there on the sidebar.
The first is the code to remove the additional information tab on the single product display page:
add_filter( 'woocommerce_product_tabs', 'huyhoa_remove_additional_information', 98 ); function huyhoa_remove_additional_information( $tabs ) { unset( $tabs['additional_information'] ); return $tabs; }
Next, use this code to create a shortcode that displays all his product attributes.
if ( ! function_exists( 'product_ai_shortcode' ) ) { function product_ai_shortcode($atts) { // Shortcode attribute (or argument) $atts = shortcode_atts( array( 'id' => '' ), $atts, 'product_ai' ); // If the "id" argument is not defined, we try to get the post Id if ( ! ( ! empty($atts['id']) && $atts['id'] > 0 ) ) { $atts['id'] = get_the_id(); } // We check that the "id" argument is a product id if ( get_post_type($atts['id']) === 'product' ) { $product = wc_get_product($atts['id']); } // If not we exit else { return; } ob_start(); // Start buffering do_action( 'woocommerce_product_additional_information', $product ); return ob_get_clean(); // Return the buffered outpout } add_shortcode('product_additional_information', 'product_ai_shortcode'); }
SHORTCODE USAGE
Insert this shortcode anywhere on your Woocommerce page with a defined product id:
[product_ai id='72']
Or in php:
echo do_shortcode("[product_ai id='72']");
In a single product page (where the “additional information” product tab is removed):
[product_ai]
Or in PHP:
echo do_shortcode("[product_ai]");
You will get something like this:
Replace WooCommerce variable products price range with the min price
The code below will replace the variable price range with “Price from” with the lowest price:
add_filter( 'woocommerce_variable_sale_price_html', 'huyhoa_replace_price_range', 10, 2 ); add_filter( 'woocommerce_variable_price_html', 'huyhoa_replace_price_range', 10, 2 ); function huyhoa_replace_price_range( $price_html, $product ) { $prefix = __('Price from', 'woocommerce'); $min_price = $product->get_variation_price( 'min', true ); return $prefix . ' ' . wc_price( $min_price ); }
Replace the Variable Price range with the chosen variation price in WooCommerce 4+
The code below will update the displayed price based on the variations the customer selects from the list of product variations:
add_action('woocommerce_before_add_to_cart_form', 'huyhoa_replace_variable_price_range_with_chosen'); function huyhoa_replace_variable_price_range_with_chosen(){ global $product; if( $product->is_type('variable') ): ?><style> .woocommerce-variation-price {display:none;} </style> <script> jQuery(function($) { var p = 'p.price' q = $(p).html(); $('form.cart').on('show_variation', function( event, data ) { if ( data.price_html ) { $(p).html(data.price_html); } }).on('hide_variation', function( event ) { $(p).html(q); }); }); </script> <?php endif; }
Add Prefix / Suffix to WooCommerce Product Prices
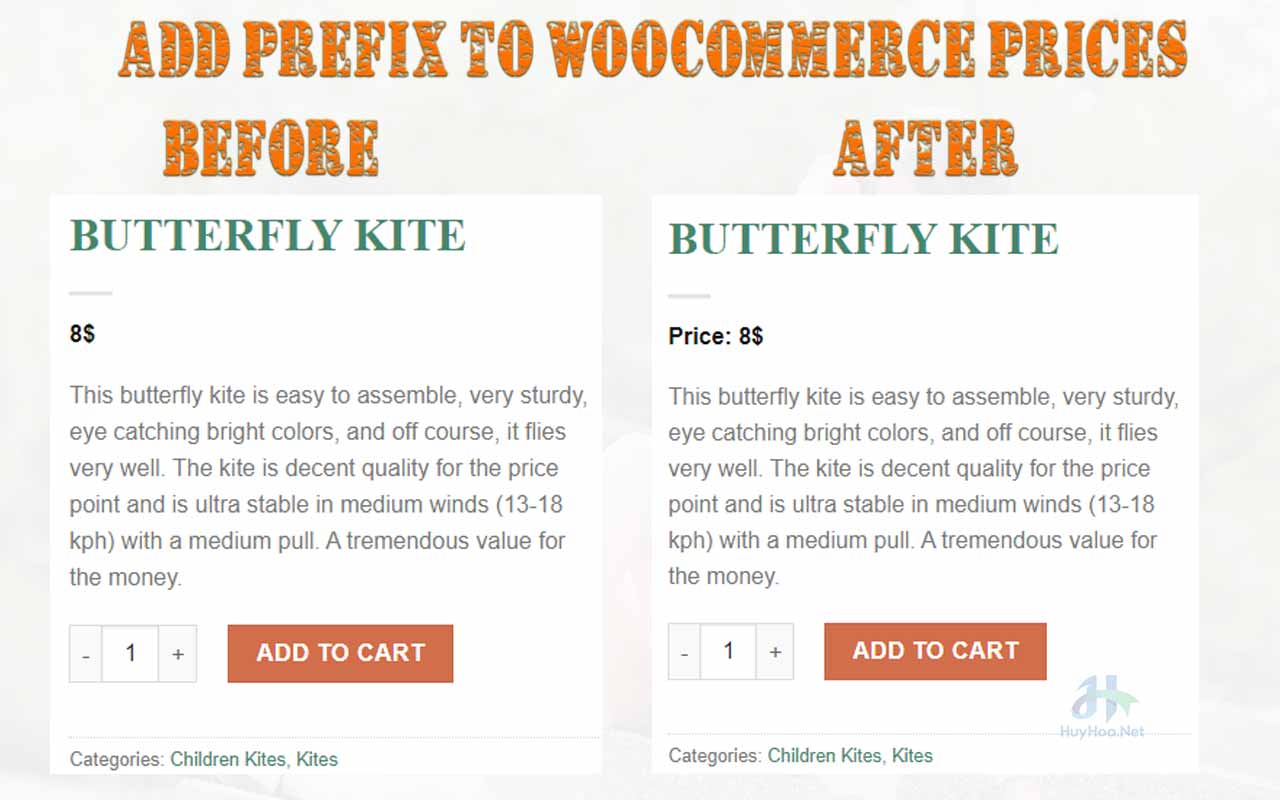
By default, WooCommerce Product Prices only shows the price in numbers and currency codes. Example $8. If you want to add any text to this price, you can use the code below. This code will convert $8 to Price: $8
add_filter( 'woocommerce_get_price_html', 'huyhoa_add_price_prefix', 99, 2 ); function huyhoa_add_price_prefix( $price, $product ){ $price = 'Price: ' . $price; return $price; }
In case you want to add to the back of the price, for example, $8 – The price includes VAT, you can refer to this code:
add_filter( 'woocommerce_get_price_html', 'huyhoa_add_after_price', 99, 2 ); function huyhoa_add_after_price( $price, $product ){ $price = $price.' - The price included VAT'; return $price; }
Result:
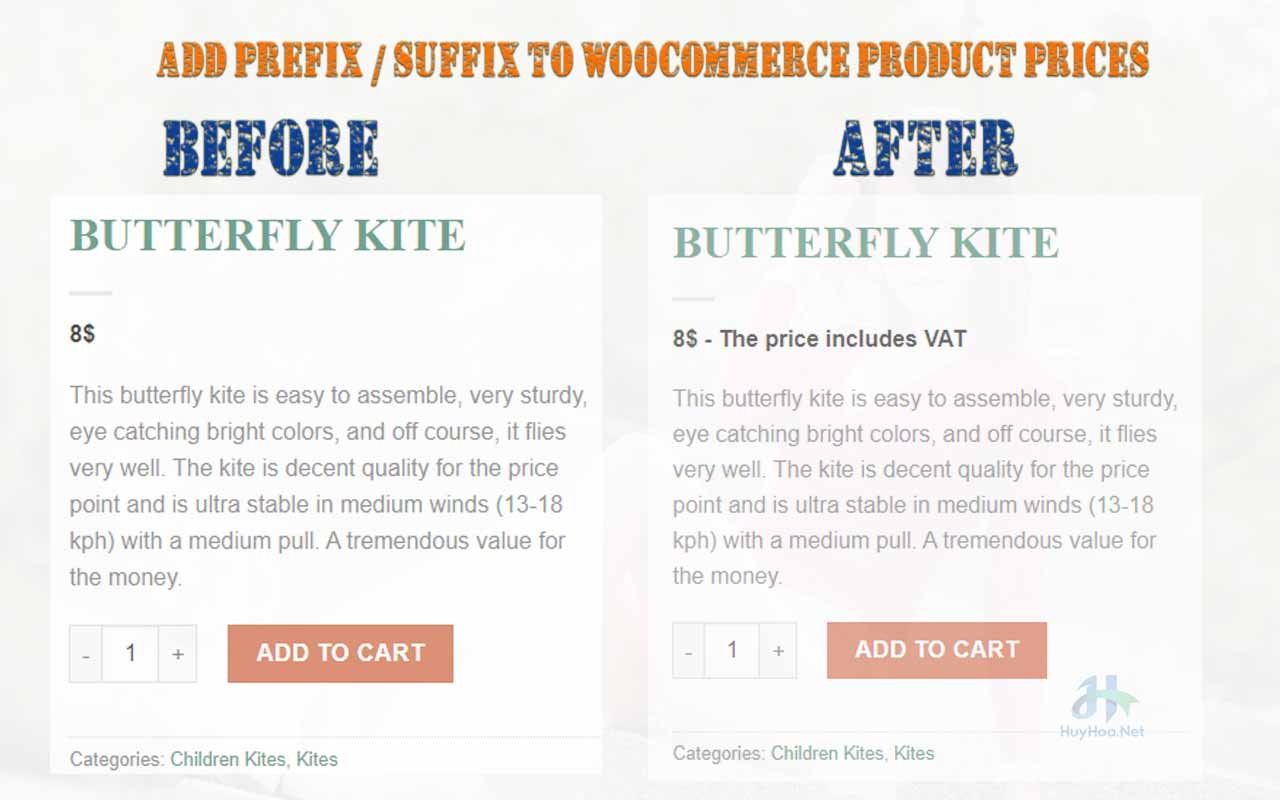
Hide Prices on the Shop & Category Pages
Remove Prices from the Shop Page Only
remove_action( 'woocommerce_after_shop_loop_item_title', 'woocommerce_template_loop_price', 10 );
Or
add_filter( 'woocommerce_get_price_html', 'huyhoa_remove_price_from_shop'); function huyhoa_remove_price_from_shop($price) { if(is_shop()) { if ( is_admin() ) return $price; return ; } else { return $price; } }
If we want all archive pages like the shop page, category page, tag page to hide price, then we will return empty price if it is not a single product page. This code ensures that prices are still displayed on single product pages, shopping cart pages, and checkout pages
function huyhoa_hide_prices_on_all_categories( $price, $product ) { if (is_product() || is_cart() || is_checkout()) { return $price; } else { return ''; } } add_filter( 'woocommerce_get_price_html', 'huyhoa_hide_prices_on_all_categories', 10, 2 );
Hide prices on WooCommerce categories archive pages
Similar to the code above, this code checks if it is a WooCommerce category archive page, it will not display the price.
function huyhoa_remove_price_from_category( $price, $product ) { if ( is_product_category() ) { return ''; // Empty string = no price! } return $price; } add_filter( 'woocommerce_get_price_html', 'huyhoa_remove_price_from_category', 10, 2 );
Hide Prices in WooCommerce for Specific Categories
Let’s say you only want to hide prices for a few categories, you can use:
add_filter( 'woocommerce_get_price_html','huyhoa_hide_price_on_taxonomy'); function huyhoa_hide_price_on_taxonomy( $price) { global $product; $hide_for_categories = array( 'adidas-shoes' ); // Hide for these category slugs / IDs if ( has_term( $hide_for_categories, 'product_cat', $product->get_id() ) ) { // Don't show price when it's in one of the categories $price= ''; } return $price; // Return original price }
Hide Prices in WooCommerce for Specific Products
Let’s say you want to hide prices for specific items with IDs 20,50,80. With the following code, you can hide the prices of products with ID 20,50,80 and show the prices for all remaining products on your Woocommerce page.
add_filter( 'woocommerce_get_price_html', 'huyhoa_hide_price_by_id', 10, 2 ); function huyhoa_hide_price_by_id( $price, $product ) { global $product; $hide_for_products_with_these_id = array( 20, 50,80 ); if ( in_array( $product->get_id(), $hide_for_products_with_these_id ) ) { return ''; // Empty string = no price! } else { return $price; // Return price for the all the other products } }
Remove the “Default Sorting” Dropdown
remove_action( 'woocommerce_before_shop_loop', 'woocommerce_catalog_ordering', 30 );
Remove or Rename SALE! Badge
When a product has a sale, Woocommerce will display a badge “Sale!” right under the product (or it could be in the product image, depending on the theme you’re using). I will guide you to Remove or Rename this SALE! Badges
Remove Sale! Label From Products – WooCommerce Shop & Single Product Pages
remove_action( 'woocommerce_before_shop_loop_item_title', 'woocommerce_show_product_loop_sale_flash', 10 ); remove_action( 'woocommerce_before_single_product_summary', 'woocommerce_show_product_sale_flash', 10 ); add_filter( 'woocommerce_sale_flash', '__return_null' );
Rename / Translate Sale! Label
add_filter( 'woocommerce_sale_flash', 'huyhoa_rename_sale_badge', 9999 ); function huyhoa_rename_sale_badge() { return '<span class="salesoff">Sale Off</span>'; // Change Sale! badge to Sale Off }
Replace “Choose an option” Text with Custom Text
This code is applied on a product page with product variations. This will make it clearer what product options your customers can choose from:
function huyhoa_change_dropdown_attribute_args( $array ) { $attribute_name = wc_attribute_label($array['attribute']); $select_text = 'Select ' . $attribute_name; $array['show_option_none'] = __( $select_text, 'WooCommerce' ); return $array; }; add_filter( 'WooCommerce_dropdown_variation_attribute_options_args', 'huyhoa_change_dropdown_attribute_args', 10, 1 );
Change “Total” text on WooCommerce Order confirmation template
function huyhoa_rename_total( $translated_text, $untranslated_text, $domain ) { if( !is_admin()) { if( $untranslated_text == 'Total:' ) $translated_text = __( 'Total excl VAT:','huyhoa' ); } if( !is_admin() && is_checkout() ) { if( $untranslated_text == 'Total' ) $translated_text = __( 'Total excl VAT:','huyhoa' ); } return $translated_text; } add_filter('gettext', 'huyhoa_rename_total', 20, 3);
How to Hide the “Add to Cart” button in WooCommerce the RIGHT way? If you search for the keyword “remove add to cart button Woocommerce”, you will get tons of tutorials. However, after trying the methods they guide for a while, none of them work, even causing website errors. Then I had to dig through the Woocommerce source code, and I found this code in the file single-product/add-to-cart/simple.php
On the 22nd line, you will see
global $product; if ( ! $product->is_purchasable() ) { return; }
So, to hide the “Add to Cart” button, simply set is_purchasable to false.
Then the Right solution is often Officially documented
Add the following code to your functions.php
file, and tara, the “Add to Cart” button is gone. Even if the “Add to Cart” button is inserted manually, it won’t work. Clicking on it will show a message “Sorry, this product is currently not available for purchase.”
Woocommerce hide add to cart button for specific products
Now, suppose you want to hide add to cart button for specific products in Woocommerce, you can use Conditional Tags. Here is checking the products with certain ids that we want to hide the “Add to Cart” button.
add_filter('woocommerce_is_purchasable', 'huyhoa_products_is_not_purchasable', 10, 2); function huyhoa_products_is_not_purchasable($is_purchasable, $product) { global $product; $products_hide_button = array(10,20,30); // IDs of products you want to hide the add to cart button if (in_array($product->get_id(), $products_hide_button)) { return false; } else { return $is_purchasable; } }
Alternatively, you can also completely hide the “Add to Cart” button using CSS:
.single_add_to_cart_button.button.alt { display: none; }
Adding Contact Form 7 to WooCommerce Product
If your product has variations or can be ordered according to the customer’s wishes, customizing the product according to the customer’s wishes, you will definitely need an Enquiry form.
These Woocommerce Functions below will insert a tab into a single Woocommerce product page and display the Contact Form 7 form.
Step 1: Add a filter to create a product tab
First, we will use the filter function to add a tab containing our contact form 7 to “woocommerce_product_tabs”
add_filter('woocommerce_product_tabs','wc_product_tabs_contact_form7',10,1); function wc_product_tabs_contact_form7($tabs){ $tabs['contact_form7'] = array( 'title' => __( 'Enquiry', 'woocommerce' ), 'priority' => 50, 'callback' => 'contact_form7_tab' ); return $tabs; }
as a result, it will create a new tab with the name “Enquiry” at the end. You can rename this Enquiry to whatever title you want.
Step 2: Define a callback function
Once we have created the tab, we will have to define the callback function(contact_form7_tab) that will echo the contact form.
function contact_form7_tab(){ // just paste the contact form 7 shortcode here echo do_shortcode('[contact-form-7 id="1230" title="Enquiry this product"]'); }
Here, id="1230
” is the id of contact form 7. You must replace this id with your id for the new form to appear. You can get the id of the form as shown in the image below
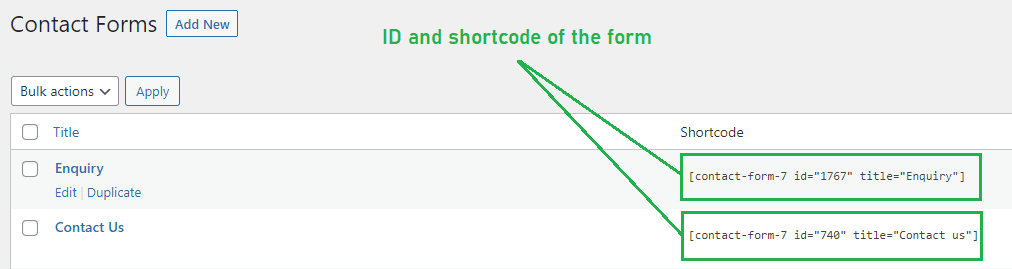
Contact Form 7 + WooCommerce – Save form data in orders
By default, Contact Form 7 will only send mail and not save data to the Database. So what if we do the product Enquiry as above, and want that form to act like a Woocommerce form? Suppose, we want when we use contact form 7 to inquiry about the product, it will be saved in the orders list of Woocommerce, is it ok?
The answer is yes, we can do it. See the code below that will do exactly that.
add_action( 'wpcf7_before_send_mail', 'convert_contact_form_order' ); function convert_contact_form_order() { global $woocommerce; $form_to_DB = WPCF7_Submission::get_instance(); if ($form_to_DB) { $formData = $form_to_DB->get_posted_data(); $your_name = $formData['your-name']; $your_email = $formData['your-email']; $your_phone = $formData['phone']; $your_address = $formData['address']; $your_message = $formData['your-message']; $product_id = $formData['product-id']; $billing_address = array( 'first_name' => $your_name, 'last_name' => $your_name, 'company' => 'N/A', 'email' => $your_email, 'phone' => $your_phone, 'address_1' => $your_address, 'address_2' => '', 'city' => 'New York', 'state' => 'NY', 'postcode' => '10020', 'country' => 'US' ); // Now we create the order $args = array( 'customer_note' => 'Customer message: '.$your_name.': '.$your_message, ); $order = wc_create_order($args); $order->add_product(get_product($product_id), 1); // Add product id and quantity $order->set_address($billing_address, 'billing' ); $shipping_method = 'COD'; $order->shipping_method_title = $shipping_method; $order->set_payment_method('check'); // You can set default payment method for this order from contact form 7 $order->calculate_totals(); $order->update_status("Processing", 'Note this order is created from Contact Form 7', true); $order->save(); } }
As you can see, that code will Save form data in orders. When using, please change the form information corresponding to the data on your contact form 7. If you have any problems, you can comment below or contact us for free help.
Add Custom Product Fields in WooCommerce
This is a tutorial on how you could add custom product fields in WooCommerce.
The code below will display 3 more fields of numeric, text, and textarea data in the General section of Product Data. When entering data and updating, the data of those 3 fields will be saved in the product’s post meta.
// Display Fields add_action('woocommerce_product_options_general_product_data', 'woocommerce_product_custom_fields'); // Save Fields add_action('woocommerce_process_product_meta', 'woocommerce_product_custom_fields_save'); function woocommerce_product_custom_fields() { global $woocommerce, $post; echo '<div class="product_custom_field">'; // HuyHoa.Net Product Text Field woocommerce_wp_text_input( array( 'id' => '_huyhoanet_product_text_field', 'placeholder' => 'HuyHoa.Net Product Text Field', 'label' => __('HuyHoa.Net Product Text Field', 'woocommerce'), 'desc_tip' => 'true' ) ); //HuyHoa.Net Product Number Field woocommerce_wp_text_input( array( 'id' => '_huyhoanet_product_number_field', 'placeholder' => 'HuyHoa.Net Product Number Field', 'label' => __('HuyHoa.Net Product Number Field', 'woocommerce'), 'type' => 'number', 'custom_attributes' => array( 'step' => 'any', 'min' => '0' ) ) ); //HuyHoa.Net Product Textarea woocommerce_wp_textarea_input( array( 'id' => '_huyhoanet_product_textarea', 'placeholder' => 'HuyHoa.Net Product Textarea', 'label' => __('HuyHoa.Net Product Textarea', 'woocommerce') ) ); echo '</div>'; } // Save all data to post meta function woocommerce_product_custom_fields_save($post_id) { // HuyHoa.Net $woocommerce_huyhoanet_text_field = $_POST['_huyhoanet_product_text_field']; if (!empty($woocommerce_huyhoanet_text_field)) update_post_meta($post_id, '_huyhoanet_product_text_field', esc_attr($woocommerce_huyhoanet_text_field)); // huyhoanet Product Number Field $woocommerce_huyhoanet_number_field = $_POST['_huyhoanet_product_number_field']; if (!empty($woocommerce_huyhoanet_number_field)) update_post_meta($post_id, '_huyhoanet_product_number_field', esc_attr($woocommerce_huyhoanet_number_field)); // huyhoanet Product Textarea Field $woocommerce_huyhoanet_textarea = $_POST['_huyhoanet_product_textarea']; if (!empty($woocommerce_huyhoanet_textarea)) update_post_meta($post_id, '_huyhoanet_product_textarea', esc_html($woocommerce_huyhoanet_textarea)); }
Now, if you want to show these fields somewhere, you just need to add the code below there.
Note: To edit the Woocommerce theme, we recommend you to work with the WooCommerce custom templates. Instructions are here
<?php // Display the value of custom product text field echo get_post_meta(get_the_ID(), '_huyhoanet_product_text_field', true); // Display the value of custom product number field echo get_post_meta(get_the_ID(), '_huyhoanet_product_number_field', true); // Display the value of custom product text area echo get_post_meta(get_the_ID(), '_huyhoanet_product_textarea', true); ?>
If outside the loop, you must declare the product ID of the product, if you leave get_the_ID() as it is, it will get the ID of the product you are viewing.
The code will look like this:
<?php // Display the value of custom product text field echo get_post_meta($product_ID, '_huyhoanet_product_text_field', true); // Display the value of custom product number field echo get_post_meta($product_ID, '_huyhoanet_product_number_field', true); // Display the value of custom product text area echo get_post_meta($product_ID, '_huyhoanet_product_textarea', true); ?>
Change Woocommerce “Thank you” message
There are many ways to change the message thanking customers for placing an order. But the best way to do this would be to filter the WC thank you message. The code below will change the default Woocommerce thank you message to
“Thank you for shopping with us on [TITLE OF WEBSITE], [NAME OF THE CUSTOMER]! We will contact you soon to confirm the order. Please pay attention to your phone number: [CUSTOMER PHONE NUMBER] to get our confirmation phone call.”
add_filter( 'woocommerce_thankyou_order_received_text', 'huyhoa_thank_you_title', 20, 2 ); function huyhoa_thank_you_title( $thank_you_title, $order ){ return 'Thank you for shopping with us on <strong>'.get_bloginfo('name').'</strong>, <font color="#d26e4b">' . $order->get_billing_last_name() . ' ' . $order->get_billing_first_name() . '</font>! We will contact you soon to confirm the order. Please pay attention to your phone number: <font color="#d26e4b">'.$order->get_billing_phone().'</font> to get our confirmation phone call.'; }
Remove billing Address at WooCommerce Checkout Page
Not only can you remove the billing address field, but you can also completely remove any field that you don’t need from the Woocommerce checkout page. The code below will remove the billing fields: postal code, company, last name, address 2 from the checkout page
add_filter( 'woocommerce_checkout_fields' , 'huyhoa_remove_billing_fields_checkout' ); function huyhoa_remove_billing_fields_checkout( $fields ) { unset($fields['billing']['billing_postcode']); unset($fields['billing']['billing_company']); unset($fields['billing']['billing_last_name']); unset($fields['billing']['billing_address_2_field']); return $fields; }
Remove Shipping address at WooCommerce Checkout Page
Similar to removing the billing fields, you can also remove the fields in the shipping area. The function below will also remove the shipping fields postal code, company, last name, address2 from the checkout page
add_filter( 'woocommerce_checkout_fields' , 'huyhoa_remove_shipping_fields_checkout' ); function huyhoa_remove_shipping_fields_checkout( $fields ) { unset($fields['shipping']['shipping_postcode']); unset($fields['shipping']['shipping_company']); unset($fields['shipping']['shipping_postcode']); unset($fields['shipping']['shipping_address_2_field']); return $fields; }
Reorder Checkout Fields in WooCommerce
Not only can you remove fields from billing and shipping, but you can also change the order of these fields. By default, Woocommerce sorts First Name -> Last Name -> Company -> Country …
The code snippet below will change the order of the billing fields. The lower the priority, the higher the order is. The example below is the email field that will be first.
add_filter( 'woocommerce_billing_fields', 'huyhoa_move_checkout_field' ); function huyhoa_move_checkout_field( $address_fields ) { $address_fields['billing_email']['priority'] = 10; $address_fields['billing_last_name']['priority'] = 11; $address_fields['billing_phone']['priority'] = 13; $address_fields['billing_first_name']['priority'] = 14; $address_fields['billing_address_1']['priority'] = 23; $address_fields['billing_city']['priority'] = 24; $address_fields['billing_address_2_field']['priority'] = 98; $address_fields['billing_country']['priority'] = 99; return $address_fields; }
Add Attributes to Short Description in WooCommerce
This Woocommerce function snippet will help to display a list of all the attributes with their value just before the short description of the single product page.
add_filter( 'woocommerce_short_description', 'add_text_in_excerpt_single_product', 1, 1 ); function add_text_in_excerpt_single_product( $post_excerpt ) { if ( is_product() ) { global $product; wc_display_product_attributes( $product ); } return $post_excerpt; }
Or the function below will show attributes right after the short description
add_action ( 'woocommerce_product_meta_start', 'show_attributes', 25 ); function show_attributes() { global $product; wc_display_product_attributes( $product ); }
Hide SKUs on WooCommerce Product Page
There are quite a few ways to disable SKUs on product pages. You can hide the display, delete the display outside the frontend but keep it in the admin, or completely remove the SKU function both in the frontend and in the admin.
SKUs are located in the General Tab of the product data when creating or editing a product. By default, the SKU is displayed on the product page directly in front of the product meta.
Remove WooCommerce SKUs Completely
If you don’t need to use SKUs at all, you can disable them entirely using this snippet in your theme’s functions.php file:
add_filter( 'wc_product_sku_enabled', '__return_false' );
Remove WooCommerce SKUs only on Product Pages
In case you just want to hide or delete SKU information outside the frontend on product pages but still keep it in the management section, we can use 2 ways. The first way is to use CSS to hide the SKU block. The second way is to use a piece of code inserted into the functions.php file in your child theme (or theme if you are not using a child theme).
Disable SKUs completely on product pages using CSS
.sku_wrapper { display: none!important; }
Disable SKUs by code snippet in functions.php
function huyhoa_remove_SKU_from_product_page( $enabled ) { if ( ! is_admin() && is_product() ) { return false; } return $enabled; } add_filter( 'wc_product_sku_enabled', 'huyhoa_remove_SKU_from_product_page' );
Disable/hide Woocommerce single product page
For some reason you don’t want product A with ID 234 to be invisible or inaccessible, you can use the code below to insert it into the functions.php file in your child theme. The function of this code is that when accessing the single product page of the product with ID 234, it will be redirected to the homepage.
add_action('wp','prevent_access_to_product_page'); function prevent_access_to_product_page(){ global $post; if ( is_product() && $post->ID === 234 ) { wp_redirect( site_url() );//will redirect to home page } }
Disable/hide Woocommerce category page
If you want to hide category pages. for example, you don’t need these because you are using category shortcode to display products on other pages. And you want when clicking on any category it will go to the Shop page, below is the code that does that function.
add_action( 'template_redirect', 'wc_redirect_to_shop'); function wc_redirect_to_shop() { // Only on product category archive pages (redirect to shop) if ( is_product_category() ) { wp_redirect( wc_get_page_permalink( 'shop' ) ); exit(); } }
Get custom product attributes in Woocommerce
This code shows a single attribute of the product. You can rely on it to choose to display several attributes of a product or create shortcodes to display elsewhere as you like.
function show_single_attributes() { global $product; $koostis = $product->get_attribute('pa_your-single-attribute'); return $koostis; }
The function below will show the contact us button and a link to the /contact-us/ page if the product price is empty or = 0. Other products with a price will still display the price and the Add to cart button as usual.
add_filter( 'woocommerce_get_price_html', 'bbloomer_price_free_zero', 9999, 2 ); function bbloomer_price_free_zero( $price, $product ) { if ( $product->is_type( 'variable' ) ) { $prices = $product->get_variation_prices( true ); $min_price = current( $prices['price'] ); if ( 0 == $min_price ) { $max_price = end( $prices['price'] ); $min_reg_price = current( $prices['regular_price'] ); $max_reg_price = end( $prices['regular_price'] ); if ( $min_price !== $max_price ) { $price = wc_format_price_range( 'FREE', $max_price ); $price .= $product->get_price_suffix(); } elseif ( $product->is_on_sale() && $min_reg_price === $max_reg_price ) { $price = wc_format_sale_price( wc_price( $max_reg_price ), 'FREE' ); $price .= $product->get_price_suffix(); } else { $price = 'FREE'; } } } elseif ( 0 == $product->get_price() || NULL == $product->get_price() ) { $price = '<span class="woocommerce-Price-amount amount">FREE</span>'; } return $price; }
Add custom fields to WooCommerce registration form
The complete code below will Add Woocommerce registration form custom field using woocommerce_forms_field filter hook and save data with nonce verification.
/* * Adding more fields to Woocommerce Registration form and My account page */ function huyhoa_woocommerce_registration_form_fields() { return apply_filters( 'woocommerce_forms_field', array( 'billing_vatnumber' => array( 'type' => 'text', 'label' => __( 'VAT Number', 'huyhoa' ), 'required' => false, ), 'huyhoa_user_howfind' => array( 'type' => 'select', 'label' => __( 'How do you find us?', 'huyhoa' ), 'options' => array( '' => __( 'Select', 'huyhoa' ), 'Adwords' => __( 'Adwords', 'huyhoa' ), 'Pinterest' => __( 'Pinterest', 'huyhoa' ), 'Facebook' => __( 'Facebook', 'huyhoa' ), 'Instagram' => __( 'Instagram', 'huyhoa' ), 'Linkedin' => __( 'Linkedin', 'huyhoa' ), 'Organic Search' => __( 'Organic Search', 'huyhoa' ), 'Other' => __( 'Other', 'huyhoa' ), ) ) ) ); } function huyhoa_woocommerce_edit_registration_form() { $fields = huyhoa_woocommerce_registration_form_fields(); foreach ( $fields as $key => $field_args ) { woocommerce_form_field( $key, $field_args ); } } add_action( 'woocommerce_register_form', 'huyhoa_woocommerce_edit_registration_form', 15 ); /** * Save registration form custom fields */ function huyhoa_save_extra_register_fields( $customer_id ) { if( wp_verify_nonce( sanitize_text_field( $_REQUEST['woocommerce-register-nonce'] ), 'woocommerce-register' ) ) { if ( isset( $_POST['billing_vatnumber'] ) ) { update_user_meta( $customer_id, 'billing_vatnumber', sanitize_text_field( $_POST['billing_vatnumber'] ) ); update_user_meta( $customer_id, 'shipping_vatnumber', sanitize_text_field( $_POST['billing_vatnumber'] ) ); } if ( isset( $_POST['huyhoa_user_howfind'] ) ) { update_user_meta( $customer_id, 'huyhoa_user_howfind', sanitize_text_field( $_POST['huyhoa_user_howfind'] ) ); } } } add_action( 'woocommerce_created_customer', 'huyhoa_save_extra_register_fields' );
WooCommerce show prices after login
You won’t need to use the Show Prices After Login Plugin for WooCommerce. The code below will make WooCommerce show prices after login
add_action( 'init', 'shessvy_hide_price_add_cart_not_logged_in' ); function shessvy_hide_price_add_cart_not_logged_in() { if ( ! is_user_logged_in() ) { add_filter( 'woocommerce_is_purchasable', '__return_false'); add_action( 'woocommerce_single_product_summary', 'shessvy_print_login_to_see', 31 ); add_action( 'woocommerce_after_shop_loop_item', 'shessvy_print_login_to_see', 11 ); add_action( 'woocommerce_simple_add_to_cart', 'shessvy_print_login_to_see', 30 ); } } function shessvy_print_login_to_see() { echo '<a href="' . get_permalink(wc_get_page_id('myaccount')) . '" rel="nofollow ugc">' . __('Login to see prices', 'theme_name') . '</a>'; }
The code above will completely block the price view or show the Add to Cart button for customers who are not logged in. Even if you use the add-to-cart=ID URL, the system will notify you to log in or register an account before adding to the cart or viewing the price.