woocommerce_form_field() is a function that outputs the payment/address form field in the Woocomerce plugin.
Table of Contents
Information
Function name: woocommerce_form_field
Plugin ref: WooCommerce
Source file: woocommerce/includes/wc-template-functions.php
Called by: woocommerce/templates/checkout/form-billing.php
, woocommerce/templates/checkout/form-shipping.php
, woocommerce/templates/myaccount/form-edit-address.php
Parameters
$key: ( string ) required – Key.
$args: ( mixed ) required – Arguments.
$value: ( string ) optional – (default: null).
$args – Array of field parameters
$args parameters include the following:
type: woocommerce_form_field helps you to create fields of different types. Below is a list of the types of HTML fields it can generate. Surely I don’t need to explain more about these default HTML field types like <input type="number" />
anymore, do I?.
Here is the list that this function supports
- text
- select
- radio
- password
- datetime
- datetime-local
- date
- month
- time
- week
- number
- URL
- tel
- country – a dropdown list of countries supported by your store, which are set in WooCommerce settings > General tab. The interesting thing is that depending on the field
$key
parameter it will display a different list of countries. So, if you pass the value of the field equal to shipping_country it will display the countries you ship to (another WooCommerce setting). - state – a dropdown list of states.
country (string): Only for state field types. Name of a country which states you would like to display. If not passed, this field will try to get states of a billing or shipping country.
label (string): Field label.
description (string): Any custom text (HTML tags are supported too) will be displayed just under а field.
placeholder (string): You can use it to add a placeholder to any supported fields like text and textarea.
maxlength (int): Adds maxlength HTML attribute to the field. Defaults to false (no attribute added).
required (bool): Do you think it adds the required HTML attribute? Example: <input type="text" required="required" />
No, it doesn’t! All this parameter does is add an asterisk near the field label. If you want to add the required attribute to the input field then read Add an attribute to woocommerce_form_field below
Custom your checkout field with woocommerce_form_field
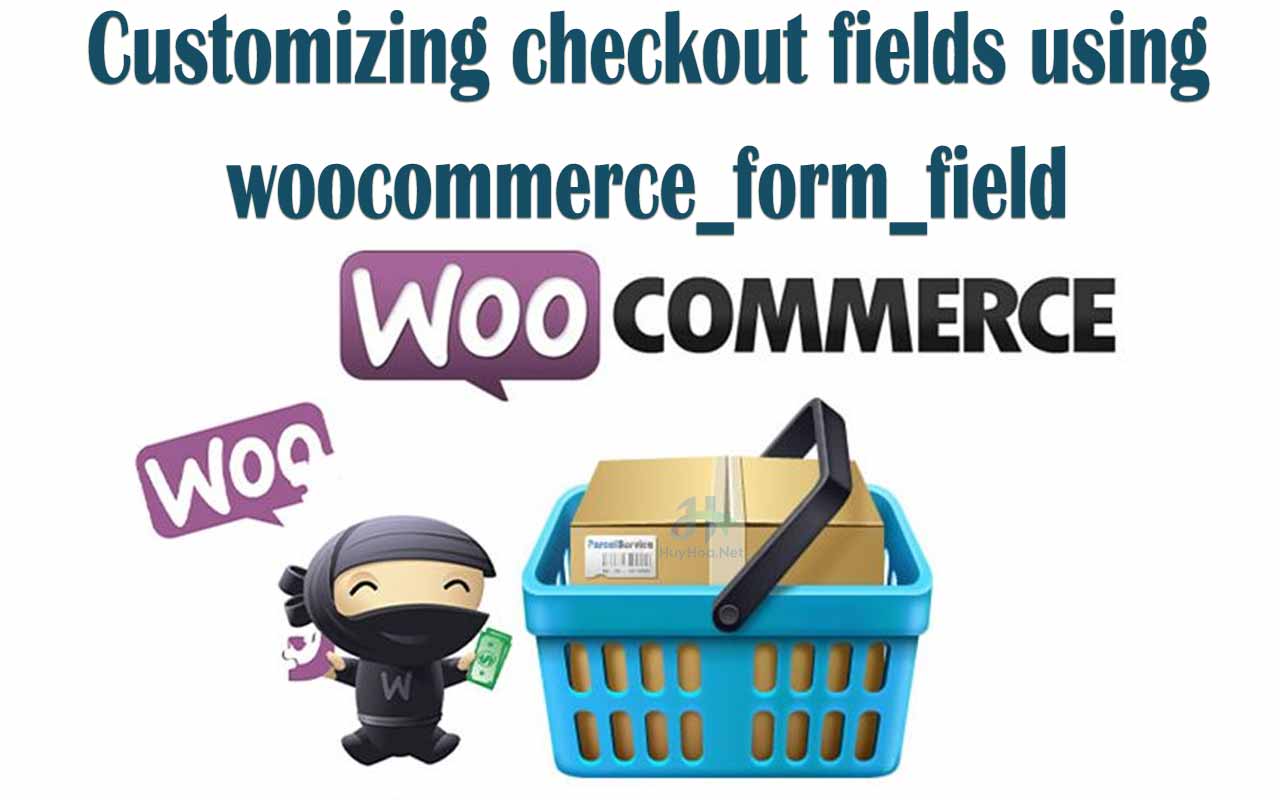
1 |
$string = woocommerce_form_field( $key, $args, $value ); |
Some examples of how to use the woocommerce_form_field() function
Display a VAT number for the B2B field on the Checkout Page
Add this code snippet to functions.php in your theme. It will add a VAT number for the B2B field on Checkout Page.
1 2 3 4 5 6 7 8 9 10 |
add_action( 'woocommerce_after_checkout_billing_form', 'add_vat_field_to_checkout' ); function add_vat_field_to_checkout() { woocommerce_form_field( 'vat', array( 'type' => 'text', 'required' => true, 'label' => 'VAT number', 'description' => 'Please enter your VAT number', ), $checkout->get_value( 'vat' ) ); } |
And the result of the code:
woocommerce_form_field() select options
This function Use woocommerce_form_field() to add a custom select options field to the check-out page
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
add_action( 'woocommerce_before_order_notes', 'huyhoa_select_checkout_field' ); function huyhoa_select_checkout_field( $checkout ) { woocommerce_form_field( 'contactmethod', array( 'type' => 'select', 'required' => 'true', 'class' => array('contactmethod-class form-row-wide'), 'label' => __( 'How can we contact you?'), 'options' => array( // options for <select> or <input type="radio" /> '' => 'Please select', // empty values means that field is not selected 'via-email' => 'Via Email', // 'value'=>'Name' 'via-telephone' => 'Via Telephone', 'via-whatsup' => 'Via Whatsup') ), $checkout->get_value( 'contactmethod' )); } |
Add custom CSS class to WooCommerce checkout fields
I would like to share the way I used to add a custom CSS class to WooCommerce checkout fields. I’m using Twitter Bootstrap 4.7 and I would like to be able to use their .form-control class. Here I will use the PHP foreach function to run through each form field then add the value to the class
1 2 3 4 5 6 7 8 9 10 11 12 13 |
add_filter('woocommerce_checkout_fields', 'huyhoa_custom_css_class_ToCheckoutFields' ); function huyhoa_custom_css_class_ToCheckoutFields($fields) { foreach ($fields as &$fieldset) { foreach ($fieldset as &$field) { // if you want to add the form-group class around the label and the input $field['class'][] = 'form-group'; // add form-control to the actual input $field['input_class'][] = 'form-control'; } } return $fields; } |
‘type’ => ‘hidden’ is not supported by woocommerce_form_field(). Therefore, we must use our own function to display and save the data of this hidden field when the customer submits the order.
The example below will save the IP address of the customer when submitting the order to the database along with the order data
1 2 3 4 5 6 7 8 9 |
add_action( 'woocommerce_after_order_notes', 'my_custom_checkout_hidden_field', 10, 1 ); function my_custom_checkout_hidden_field( $checkout ) { $user_ip = $_SERVER['REMOTE_ADDR']; // Output the hidden link echo '<div id="user_link_hidden_checkout_field"> <input type="hidden" class="input-hidden" name="user_ip" id="user_ip" value="' . $user_ip . '"> </div>'; } |
Then you will need to save this hidden field in the order:
1 2 3 4 5 6 |
add_action( 'woocommerce_checkout_update_order_meta', 'save_custom_checkout_hidden_field', 10, 1 ); function save_custom_checkout_hidden_field( $order_id ) { if ( ! empty( $_POST['user_ip'] ) ) update_post_meta( $order_id, '_user_ip', sanitize_text_field( $_POST['user_ip'] ) ); } |
Add checkbox field to the checkout
The code below has the goal of adding a checkbox that says “Add me to your mailing list”. This functionality does work to provide a checkbox that is required to proceed.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
// Add a checkbox field to the WooCommerce checkout page add_action( 'woocommerce_register_form', 'huyhoa_add_checkbox_to_checkout', 11 ); function huyhoa_add_checkbox_to_checkout() { woocommerce_form_field( 'receive_email', array( 'type' => 'checkbox', 'class' => array('form-row receive-email'), 'label_class' => array('woocommerce-form__label woocommerce-form__label-for-checkbox checkbox'), 'input_class' => array('woocommerce-form__input woocommerce-form__input-checkbox input-checkbox'), 'required' => true, 'label' => 'I want to receive email from Huy Hoa', )); } // Show error after clicking submit button if the user has not checked checkbox add_filter( 'woocommerce_registration_errors', 'hh_validate_receive_email', 10, 3 ); function hh_validate_receive_email( $errors, $username, $email ) { if ( ! is_checkout() ) { if ( ! (int) isset( $_POST['receive_email'] ) ) { $errors->add( 'receive_email_reg_error', __( 'You are not agree to receive email from us!', 'woocommerce' ) ); } } return $errors; } |
If you want the Checkbox Checked by default, you can replace the functions below in the code above:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
add_action( 'woocommerce_register_form', 'huyhoa_add_checkbox_to_checkout', 11 ); function huyhoa_add_checkbox_to_checkout() { $checked = $checkout->get_value( 'receive_email' ) ? $checkout->get_value( 'receive_email' ) : 1; echo '<div id="my-new-field"><h3>'.__('I want to receive email from Huy Hoa: ').'</h3>'; woocommerce_form_field( 'receive_email', array( 'type' => 'checkbox', 'class' => array('input-checkbox'), 'label' => __('I want to receive email from Huy Hoa.'), 'required' => true, ), $checked); echo '</div>'; } |
Add an attribute to woocommerce_form_field
If you actually want to add an attribute to woocommerce_form_field then you can add the woocommerce_form_field_checkbox filter hook.
You just need to add this hook after the code you added the custom field
1 2 3 4 5 6 7 8 |
function filter_woocommerce_form_field_checkbox( $field, $key, $args, $value ) { // Based on key if ( $key == 'receive_email' ) { $field = str_replace( '<input', '<input required', $field ); } return $field; } add_filter( 'woocommerce_form_field_checkbox', 'filter_woocommerce_form_field_checkbox', 10, 4 ); |
As you can see, the example above will require the receive_email field to be required. However, in my opinion, the required parameter is a bit disappointing. You would always expect that WooCommerce developers to at least implement both, must validate required ” both in frontend and backend. However, currently, if you want to “required” a certain field, you need to build an accompanying function to validate it.
Set default value of woocommerce_form_field
To add a default value arg to the checkout form fields, simply add ‘default’ => $value, to the array in the function. Eg:
1 2 3 4 5 6 7 |
woocommerce_form_field( 'how_old', array( 'type' => 'text', 'required' => 'true', 'default' => 25, // Default value show 25 'class' => array('howold-class form-row-wide'), 'label' => __('How old are you?'), ), $checkout->get_value( 'how_old' )); |
Woocommerce_form_field radio
Add radio buttons before the order total in the WooCommerce Order review section. I figured out how to add custom radio buttons to the order review section. Here is the code:
1 2 3 4 5 6 7 8 |
woocommerce_form_field( 'delivery', array( 'type' => 'radio', 'class' => array( 'form-row-wide', 'update_totals_on_change' ), 'options' => array( '2.95' => 'Urgent delivery: €2.95', '0' => 'Normal delivery: 24 - 48 HOURS (free)', ), ), $chosen ); |
Woocommerce_form_field priority
To set priority for custom fields or reorder checkout fields, you must first create that field
1 |
woocommerce_form_field( 'vat', array( 'type' => 'text', 'required' => true, 'label' => 'VAT number', 'description' => 'Please enter your VAT number', ), $checkout->get_value( 'vat' ) ); |
You then rearrange the order of the fields this way:
1 |
$fields['billing_vat']['priority'] = 10; |
The code above will move the position of the VAT field to the top right after the first name field
Woocommerce_form_field read-only
There are 2 ways to create a read-only field for Woocommerce Billing Fields or Woocommerce Shipping Fields
The first way is to use the Add an attribute to woocommerce_form_field as mentioned above to add the readonly=”readonly” attribute to it.
Method 2, use the code below:
1 2 3 4 5 6 7 8 9 |
add_filter( 'woocommerce_billing_fields', 'huyhoa_readonly_billing_account_fields', 25, 1 ); function huyhoa_readonly_billing_account_fields ( $billing_fields ) { // Only my account billing address for logged in users $readonly = ['readonly' => 'readonly']; $billing_fields['billing_first_name']['custom_attributes'] = $readonly; $billing_fields['billing_last_name']['custom_attributes'] = $readonly; $billing_fields['billing_email']['custom_attributes'] = $readonly; return $billing_fields; } |
Note that it does not apply to live websites because the example above will make the 3 fields first_name / last_name / email read-only and cannot enter data.
The article references some tutorials from WooCommerce Docs and Stack Overflow